Bootstrap Input Multiple Tags Using Tag Manager: Step-by-Step Guide
19 Oct 2024 01:02 PM
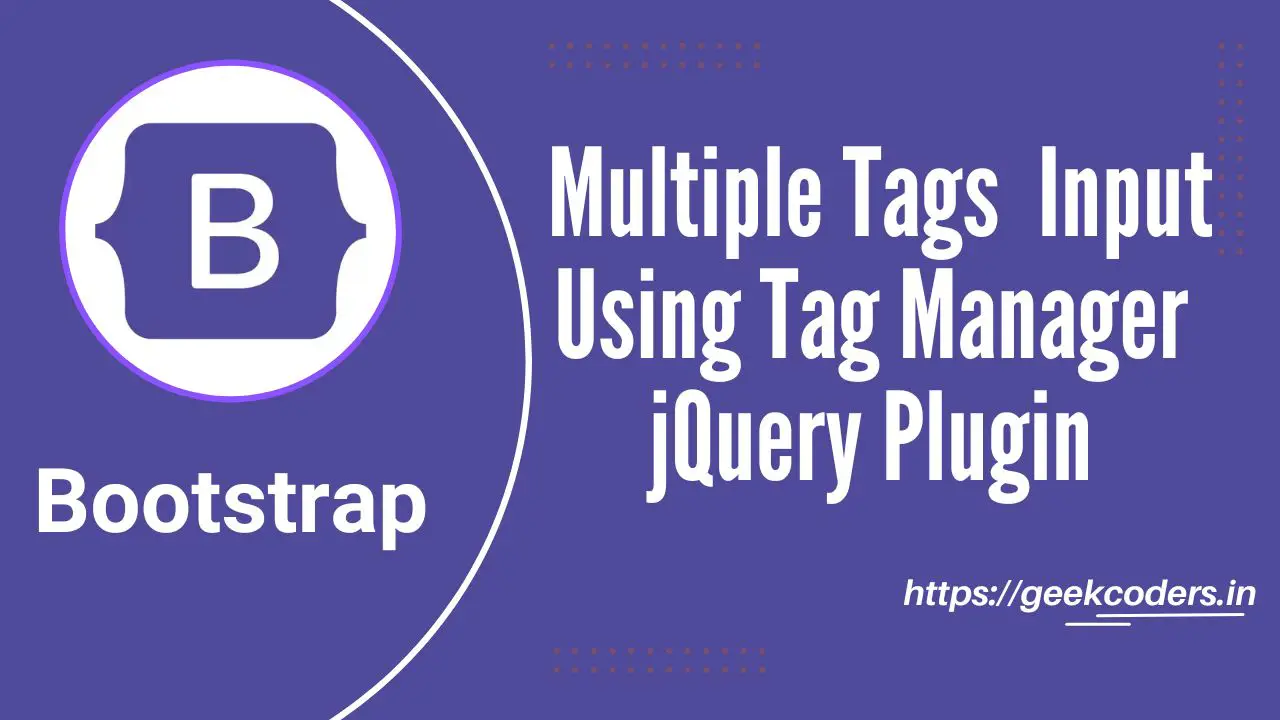
2
30