Laravel Eloquent: Best Practices for Relationships and Query Optimization
28 Jan 2025 06:40 PM
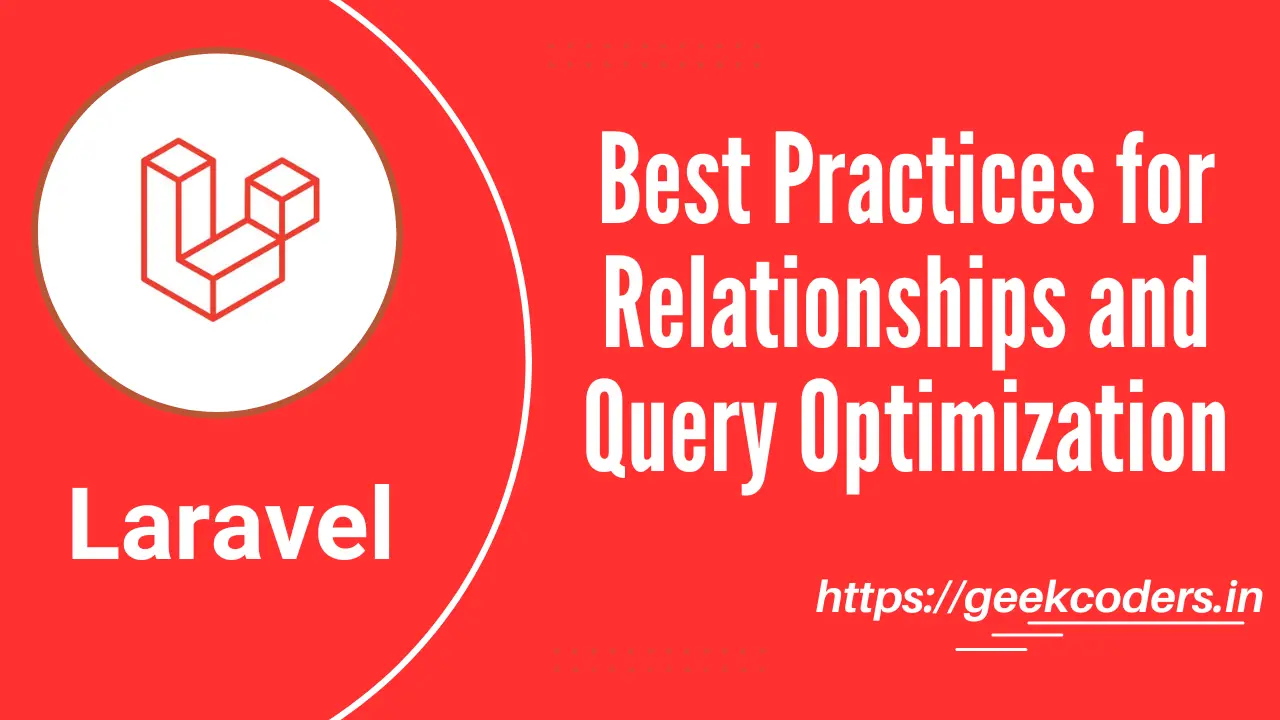
1
18